Everything you need to develop a Bar, Scatter, or Line chart **Properly** in Python!
A self-contained code repository to develop a Bar, Scatter, or Line chart using Matplotlib and Seaborn in Python
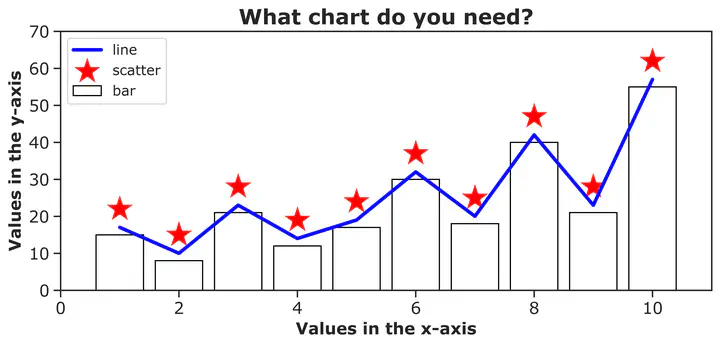
At the end of this blog, you will be able to
- Develop a Bar, Scatter, Line, or a combination of these charts just by commenting and/or un-commenting parts of codes;
- Define the usually necessary characteristics of the charts easily;
- Customize the title, labels of the axis, tickmarks in the axis, and legend easily.
Requirements:
- Basic syntaxing knowledge of Python
- Know-how to install a library in Python
During my Ph.D. life as well as in my professional career as a Senior Data Scientist at Walmart, I had to and still need to create lots of charts to visualize some input data or outputs from optimization or machine learning models. I have noticed that most of the charts I create are either a line, a scatter, or a bar chart. Like almost all data sceintist/analyst out there, I used to go to StackOverflow or Matplotlib Documentations or some other website whenever I needed to create a chart. At some point, this process of going back and forth had just become too tedious and time-consuming.
Another issue I have found while browsing for a solution to create a chart was that most sources did not contain a full-fledged chart. A chart is not just drawing a line or some bars on a white background. One need to think about correctly developing the title, axis labels, tickmarks, and texts on chart body. One also need consider resolution of the chart while saving it. These factors are as important as the lines or bars, especially if you plan to use the figure in your journal or conference papers.
To avoid these issues, I created a code repository for myself using Matplotlib in Python. The repository contains code snippets for the necessary elements to create either a line, a scatter, or a bar chart. One just need to comment or un-comment parts of the code to get their desired chart. This repository has saved me substantial amount of time and I hope it will do the same for you! See the code block below.
# Make sure the lengths of x-axis values and y-axis values match!
# Here I have defined x-axis and y-axis values for different charts
# For bar chart
x_bar = [1,2,3,4,5,6,7,8,9,10]
y_bar = [15,8,21,12,17,30,18,40,21,55]
# For line chart
x_line = [1,2,3,4,5,6,7,8,9,10]
y_line = [17,10,23,14,19,32,20,42,23,57]
# For line chart
x_scatter = [1,2,3,4,5,6,7,8,9,10]
y_scatter = [22,15,28,19,24,37,25,47,28,62]
# Import libraries
import seaborn as sns
from matplotlib import pyplot as plt
#------------------------------------------------------------------------------
# Section: Names and labels
#------------------------------------------------------------------------------
title_of_fig = "What chart do you need?"
label_of_x_axis = "Values in the x-axis"
label_of_y_axis = "Values in the y-axis"
name_of_figure_to_save_as = "drawyourownchart.jpg"
#------------------------------------------------------------------------------
# Section: Style and fonts
#------------------------------------------------------------------------------
# Define the background color of your figure
sns.set_theme(style="ticks")
# You can try other background color/styles:
# "whitegrid", "darkgrid", "dark", "white"
# Define the font family for your figure
# Using the same font family for all texts in your figure is highly recommended.
plt.rcParams['font.family'] = 'Verdana'
#------------------------------------------------------------------------------
# Section: Width and height
#------------------------------------------------------------------------------
# Define the width and height of your figure
fig_width = 10
fig_height = 4
# Create the figure object with the defined width and height
ax1 = plt.figure(figsize=(fig_width, fig_height))
#------------------------------------------------------------------------------
# Section: Bar graph
#------------------------------------------------------------------------------
# x: List of x-axis values
# y: List of y-axis values
# width: Width(s) of the bars; Any value between 0 and 1
# color: Fill-in color of the bars
# edgecolor: Color of the edges of the bars
# label : Text to show in the legend
# If you do not want to plot a bar graph, comment the following line of code
ax1 = plt.bar(x_bar, y_bar, width = 0.8, color='white',
edgecolor='black', label = 'bar')
#------------------------------------------------------------------------------
# Section: Line chart
#------------------------------------------------------------------------------
# x: List of x-axis values
# y: List of y-axis values
# linewidth: Width of the line; usually between 1 and 5
# color: Color of the line
# linestyle: Four options: "solid", "dashed", "dotted", "dashdot"
# label: Text to show in the legend
# If you do not want to plot a line chart, comment the following line of code
ax1 = plt.plot(x_line, y_line, linewidth = 3, color='blue',
linestyle = 'solid', label = 'line')
#------------------------------------------------------------------------------
# Section: Scatter plot (using Seaborn)
#------------------------------------------------------------------------------
# x: List of x-axis values
# y: List of y-axis values
# s: Size of your marker; usually between 100 and 1000
# color: Color of the marker
# linestyle: Type of markers; some options: "o", '*', 's', 'v'
# label: Text to show in the legend
# If you do not want to plot a scatter plot, comment the following line of code
ax1 = sns.scatterplot(x = x_scatter, y = y_scatter, s = 1000, color = 'red',
marker = '*', label = 'scatter')
#------------------------------------------------------------------------------
# Section: Title
#------------------------------------------------------------------------------
# fontsize: Size of font
# fontweight: 'bold', 'italic' etc.
plt.title(title_of_fig, fontsize = 18, fontweight = 'bold')
#------------------------------------------------------------------------------
# Section: X-axis
#------------------------------------------------------------------------------
# fontsize: Size of font
# fontweight: 'bold', 'italic' etc.
plt.xlabel(label_of_x_axis, fontsize = 14, fontweight = 'bold')
# Control the tick labels along the x-axis
# rotation: The angle at which you would like to show your tick labels
# fontsize: Size of font
plt.xticks(rotation = 0, fontsize = 14)
# You can control the range of x-axis values using the following line of code
plt.xlim([0,11])
#------------------------------------------------------------------------------
# Section: Y-axis
#------------------------------------------------------------------------------
# fontsize: Size of font
# fontweight: 'bold', 'italic' etc.
plt.ylabel(label_of_y_axis, fontsize = 14, fontweight = 'bold')
# Control the tick labels along the y-axis
# rotation: The angle at which you would like to show your tick labels
# fontsize: Size of font
plt.yticks(rotation = 0, fontsize = 14)
# You can control the range of y-axis values using the following line of code
plt.ylim([0,70])
#------------------------------------------------------------------------------
# Section: Legend
#------------------------------------------------------------------------------
# loc: The location of the legend in the figure; examples: "upper right",
# "upper left", "lower left", "lower right"
# prop: 'size' to increase or decrease the legend size
plt.legend(loc="upper left", prop={'size': 20})
#------------------------------------------------------------------------------
# Section: Save figure
#------------------------------------------------------------------------------
# dpi: dots per inch, the higher the number, the better the resolution.
plt.savefig(name_of_figure_to_save_as, dpi = 600)
#------------------------------------------------------------------------------
# Section: Show figure
#------------------------------------------------------------------------------
plt.show()